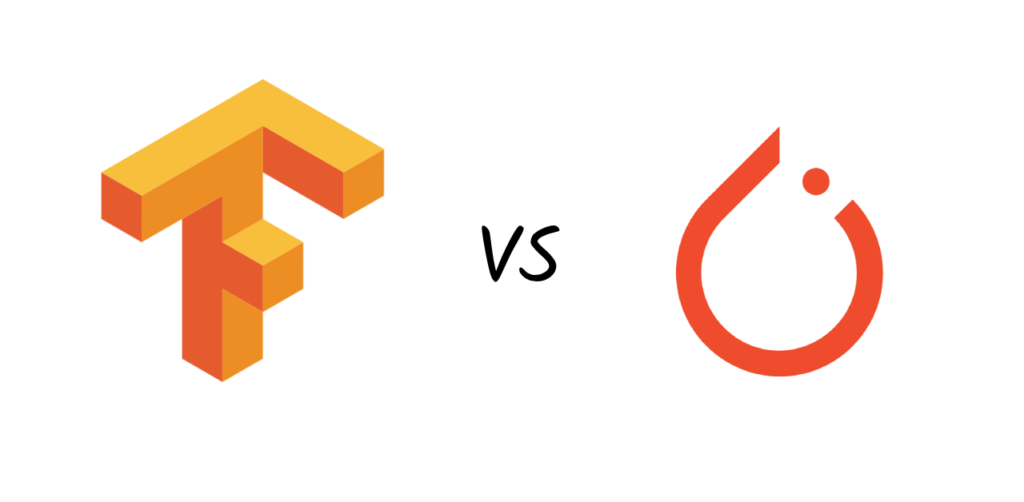
Machine learning frameworks are crucial in developing and deploying models, providing tools to streamline and optimize the process. Among the many available frameworks, TensorFlow and PyTorch are two of the most popular and widely used. Both have strengths and weaknesses, making them suitable for different projects and users. In this blog post, we will explore the pros and cons of TensorFlow and PyTorch, providing examples to illustrate their use cases.
TensorFlow
Pros of TensorFlow
- Mature Ecosystem: TensorFlow, developed by Google Brain, has been around since 2015. Its mature and extensive ecosystem includes TensorFlow Lite for mobile and embedded devices, TensorFlow Extended (TFX) for production ML pipelines, and TensorFlow.js for running models in the browser.
- High Performance: TensorFlow is optimized for high-performance operations, especially large-scale production environments. It supports distributed computing, making it suitable for training large models on multiple GPUs and TPUs.
- Flexibility: TensorFlow 2.x introduced eager execution by default, making it more intuitive and flexible for researchers and developers. It allows for immediate evaluation of operations, similar to PyTorch.
- Deployment Options: TensorFlow offers numerous deployment options, including TensorFlow Serving for model deployment in production environments, TensorFlow Lite for mobile and embedded devices, and TensorFlow.js for running models in the browser.
- Strong Community and Support: TensorFlow has a large and active community, extensive documentation, and numerous tutorials and courses. This makes it easier for beginners and advanced users to find solutions to complex problems.
Cons of TensorFlow
- Steep Learning Curve: Despite improvements in TensorFlow 2.x, the framework still has a steeper learning curve than PyTorch. The complexity of the API and the extensive ecosystem can be overwhelming for beginners.
- Verbose Syntax: TensorFlow’s syntax can be verbose and less intuitive, making code more challenging to read and write, especially for those new to machine learning.
- Debugging Challenges: Although TensorFlow 2.x supports eager execution, debugging can still be challenging compared to PyTorch, which naturally supports dynamic computation graphs.
TensorFlow Example
Below is an example of a simple neural network in TensorFlow for classifying handwritten digits from the MNIST dataset:
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.utils import to_categorical
# Load dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0
y_train, y_test = to_categorical(y_train), to_categorical(y_test)
# Build model
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train model
model.fit(x_train, y_train, epochs=5)
# Evaluate model
model.evaluate(x_test, y_test)
PyTorch
Pros of PyTorch
- Dynamic Computation Graphs: PyTorch is known for its dynamic computation graph (define-by-run), which allows for more flexibility and ease of debugging. This makes it particularly popular in the research community.
- Pythonic and Intuitive: PyTorch’s syntax is more Pythonic and intuitive, making it easier to learn and use, especially for those familiar with Python.
- Strong Community and Research Focus: PyTorch has a strong presence in the research community, with many new research papers and state-of-the-art models implemented in PyTorch.
- Native Support for Dynamic Neural Networks: PyTorch natively supports dynamic neural networks, which can change structure during runtime. This is particularly useful for certain types of neural networks, such as those used in natural language processing.
Cons of PyTorch
- Smaller Ecosystem: While PyTorch’s ecosystem is growing, it is still not as extensive as TensorFlow’s. For example, deployment tools are more limited, although TorchServe and ONNX are notable exceptions.
- Performance: PyTorch may not be as optimized as TensorFlow for certain large-scale production tasks, although this gap is narrowing with each new release.
- Less Mature for Production: Historically, PyTorch has been less mature for production deployment compared to TensorFlow, though this is changing with tools like TorchServe.
PyTorch Example
Here is an example of a similar neural network in PyTorch for the same MNIST classification task:
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
# Load dataset
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
train_dataset = datasets.MNIST(root='./data', train=True, transform=transform, download=True)
test_dataset = datasets.MNIST(root='./data', train=False, transform=transform)
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=64, shuffle=False)
# Define model
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.flatten = nn.Flatten()
self.fc1 = nn.Linear(28*28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = self.flatten(x)
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
model = SimpleNN()
# Define loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Train model
for epoch in range(5):
for data, target in train_loader:
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
# Evaluate model
correct = 0
total = 0
with torch.no_grad():
for data, target in test_loader:
output = model(data)
_, predicted = torch.max(output.data, 1)
total += target.size(0)
correct += (predicted == target).sum().item()
print(f'Accuracy: {100 * correct / total}%')
Conclusion
Both TensorFlow and PyTorch are potent tools for machine learning and deep learning, each with strengths and weaknesses. TensorFlow excels in production environments and has a more extensive ecosystem, making it suitable for large-scale deployments. On the other hand, PyTorch’s dynamic computation graphs and Pythonic syntax make it a favorite among researchers and those who prefer an intuitive and flexible framework.
When choosing between TensorFlow and PyTorch, consider your specific needs, such as the scale of deployment, ease of use, community support, and the nature of your projects. Both frameworks continually evolve, with each new release bringing them closer together regarding functionality and performance. Ultimately, the choice may come down to personal preference and the specific requirements of your machine-learning tasks.