This tutorial demonstrates Python imaplib
to extract emails from a Gmail account. The process includes setting up the Gmail account for IMAP access, connecting to the Gmail server using the imaplib module, logging in with the user’s credentials, selecting the mailbox, searching for specific emails, and fetching the email contents. The fetched emails are parsed and printed as plain text, enabling further processing or analysis. This method is particularly useful for automating email handling tasks using Python.
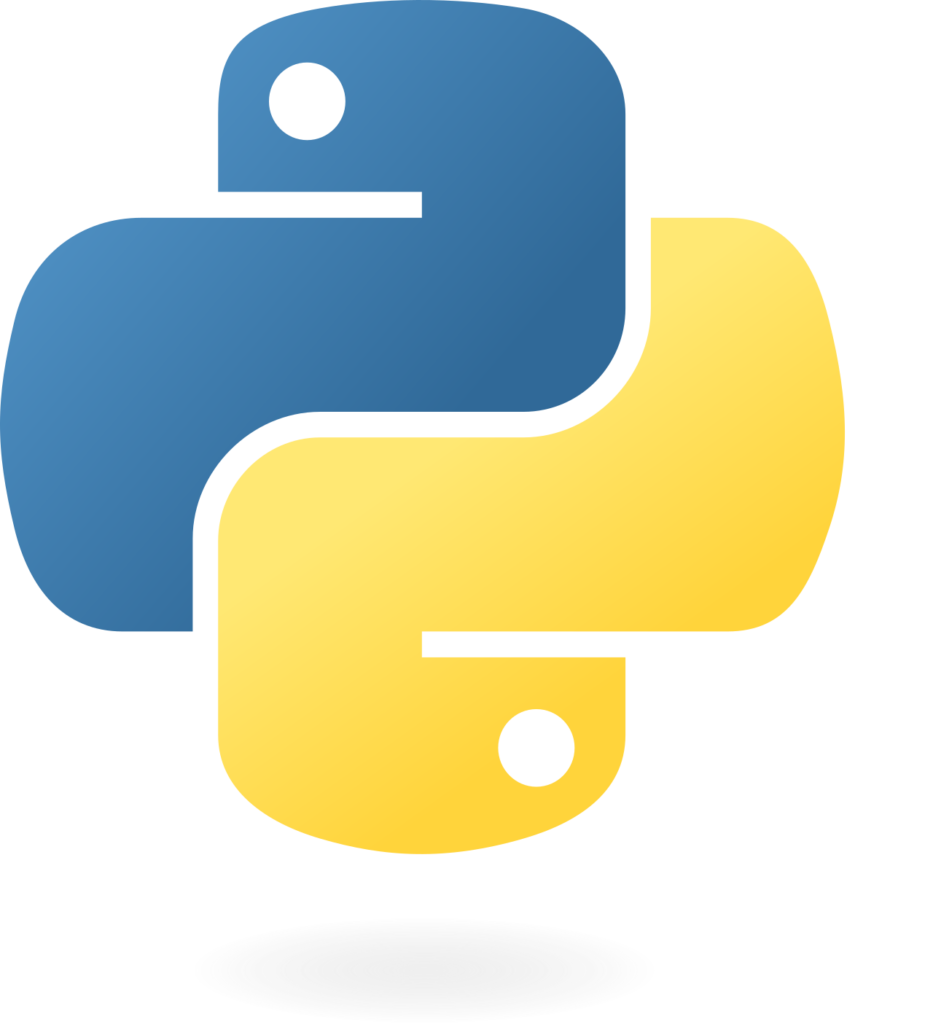
import imaplib
import email
def getDetails():
#setup the email port and give permission details
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.login('email@gmail.com', 'password')
mail.list()
mail.select('inbox')
#combs through inbox folders for the latest email
result, data = mail.uid('search', None, "ALL")
i = len(data[0].split())
for x in range(i):
latest_email_uid = data[0].split()[x]
result, email_data = mail.uid('fetch', latest_email_uid, '(RFC822)')
raw_email = email_data[0][1]
#decoding
raw_email_string = raw_email.decode('utf-8')
email_message = email.message_from_string(raw_email_string)
for part in email_message.walk():
if part.get_content_type() == "text/plain": # ignore attachments/html
body = part.get_payload(decode=True)
body = str((body.decode('utf-8')))
The above guide includes step-by-step instructions on setting up your Gmail account for IMAP access, writing Python code to connect to the Gmail server, and retrieving email contents. The post is helpful for those looking to automate email processing using Python.