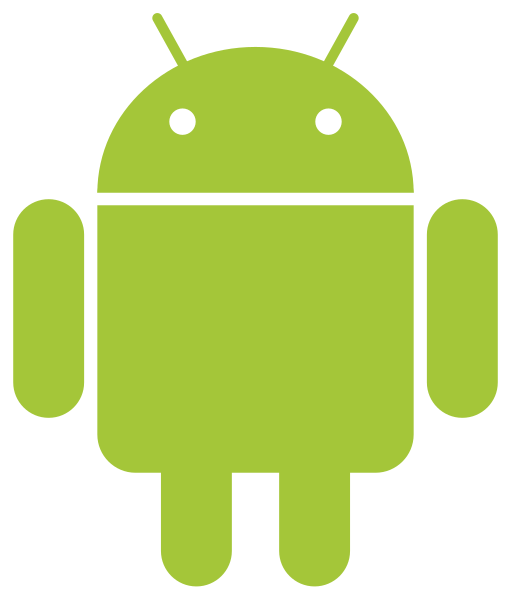
Incorporating SMS capabilities into your Android application can significantly enhance its functionality. This post delves into how you can detect incoming SMS messages and send SMS programmatically within your app. It provides step-by-step instructions, necessary permissions, and code snippets for seamless integration. Whether you’re a seasoned developer or just starting, this tutorial will equip you with the knowledge to manage SMS effectively in your Android projects.
In your fragment
val smsReciever = SmsReciever()
val smsIntentFilter = IntentFilter(Telephony.Sms.Intents.SMS_RECEIVED_ACTION)
context?.registerReceiver(smsReciever, smsIntentFilter)
In your manifest
<uses-permission android:name="android.permission.RECEIVE_SMS"/>
<uses-permission android:name="android.permission.READ_SMS"/>
In your receiver
class SmsReciever : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
val message = Telephony.Sms.Intents.getMessagesFromIntent(intent)
val content = message[0].displayMessageBody
Toast.makeText(context, content,
Toast.LENGTH_SHORT).show()
}
}
Read More about Broadcast Receiver