With the advent of HTML5, web development has taken a significant leap forward, bringing many new features and capabilities. One of the most exciting additions is the Geolocation API, which allows web developers to access a user’s location data easily. Combined with Google’s robust mapping services, this capability opens up a world of possibilities for creating location-aware web applications.
In this blog post, we’ll explore how to detect geo-location using the HTML5 Geolocation API and integrate it with Google Maps to create a dynamic, interactive map that displays the user’s current location. This tutorial assumes basic knowledge of HTML, CSS, and JavaScript.
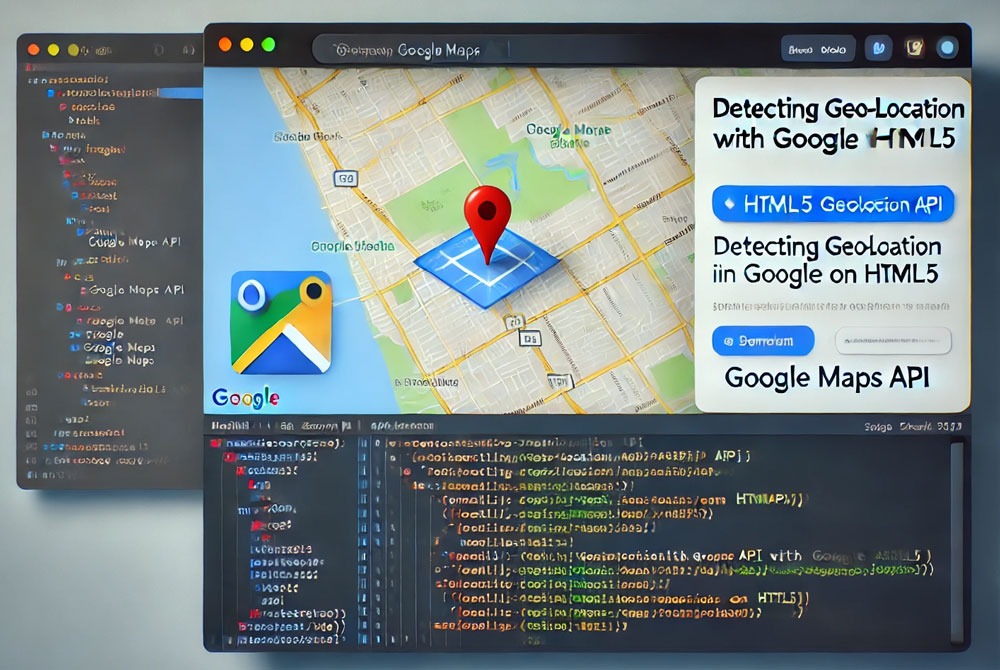
What You’ll Need
Before we get started, make sure you have the following:
- A basic HTML file setup.
- An internet connection to access the Google Maps API.
- A modern web browser that supports HTML5 Geolocation (e.g., Google Chrome, Firefox, Safari).
Step 1: Setting Up the HTML File
Let’s begin by setting up a simple HTML file. This file will include the necessary HTML, CSS, and JavaScript to display a map and detect the user’s location.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Geolocation with Google API</title>
<style>
#map {
height: 400px;
width: 100%;
}
</style>
</head>
<body>
<h1>Detecting Geo-location with Google API on HTML5</h1>
<div id="map"></div>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script>
<script>
// Your JavaScript will go here
</script>
</body>
</html>
In this code, we set up a basic HTML structure with an div
element that will hold our map. We also include a link to the Google Maps API, which requires an API key. Make sure to replace YOUR_API_KEY
with your actual Google Maps API key.
Step 2: Adding the Geolocation Script
Next, we’ll add the JavaScript code that detects the user’s location and initializes the Google Map. Add the following JavaScript within the <script>
tags in your HTML file:
function initMap() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition, showError);
} else {
alert("Geolocation is not supported by this browser.");
}
}
function showPosition(position) {
var lat = position.coords.latitude;
var lng = position.coords.longitude;
var userLocation = { lat: lat, lng: lng };
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 15,
center: userLocation
});
var marker = new google.maps.Marker({
position: userLocation,
map: map
});
}
function showError(error) {
switch(error.code) {
case error.PERMISSION_DENIED:
alert("User denied the request for Geolocation.");
break;
case error.POSITION_UNAVAILABLE:
alert("Location information is unavailable.");
break;
case error.TIMEOUT:
alert("The request to get user location timed out.");
break;
case error.UNKNOWN_ERROR:
alert("An unknown error occurred.");
break;
}
}
In this script, we define several functions:
initMap
: This function checks if the browser supports geolocation and requests the user’s current position.showPosition
: This function is called if the geolocation request is successful. It retrieves the user’s latitude and longitude, creates a Google Map centered at the user’s location, and places a marker on the map.showError
: This function handles any errors during the geolocation request and displays an appropriate message to the user.
Step 3: Testing Your Application
Save your HTML file and open it in a modern web browser. If everything is set up correctly, you should see a map centered at your current location with a marker indicating your position. If there are any issues, check the browser’s console for error messages and ensure your Google Maps API key is valid and adequately included.
Conclusion
With just a few lines of code, you can leverage the power of the HTML5 Geolocation API and Google Maps to create a dynamic, location-aware web application. This combination of technologies opens up many exciting possibilities, from simple location tracking to more complex applications like location-based services and real-time mapping.
As you explore these technologies further, consider how you might integrate additional features such as directions, location-based search, or user-generated content to create even more engaging and valuable applications. Happy coding!